In Asp.Net Core applications, Controllers have action strategies, and action methodology performs the operation once any user interacts with the interface. Suppose, once the user clicks on any button, then the corresponding action methodology gets dead however this action methodology doesn't get dead directly. it's to be passed in many steps. That means, it follows the route - once the user clicks on the button, the request is routed to seek out the Controller and also the corresponding action methodology is named.
If we would like to perform any operation before or once the action methodology is named, then we've to have faith in filters as a result of filters are used for performing arts pre- and post-logic before and once the action methodology gets dead. ASP internet Core comes with a thought of filters. Filters intercept the stages of the MVC pipeline and permit us to run code before/after their executions. they're meant to be used for cross-cutting concerns; logic that is needed across the total application, usually not business familiarized. One example is authorization wherever in a very internet API, we'd use to stop unauthorized request to execute the code in our controllers. So as to try and do that we'd have a filter at the doorway of the pipeline. In fact, ASP.Net Core has some predefine stages related to the filters.
PROCESS FLOW OF FILTERS
Filters run among the ASP.Net Core action invocation pipeline, typically remarked because of the filter pipeline. The filter pipeline runs when ASP.Net Core selects the action to execute. So, when filter executed within the pipeline, then there is always different scenarios for every execution. So, before creating a filter, we first analyze our requirements so that we can decide which filters we can require exactly and in which position of the filter pipeline for executions. In Asp.Net Core, filter always executes from the MVC Action method which is known as the Filter Pipeline and it will be executed when the action method executed.
Different filter kinds run at all completely different points along the pipeline. In the filter pipeline, some filters executed before the execution of the next level like Authorization filters. Also, there are some filters which are executed before and after the state of execution in the filter pipeline. Action filters are one of the examples of these types of filters.
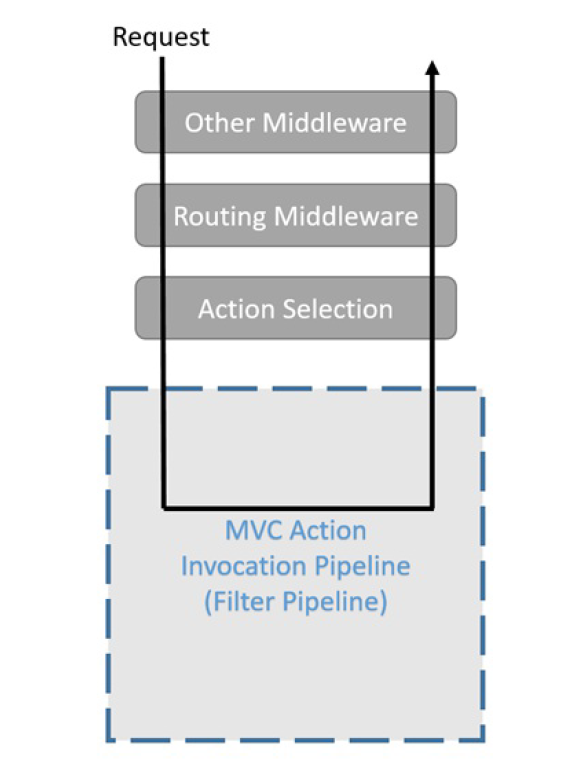
Image Source : https://bit.ly/2xePP1n
TYPES OF FILTERS
In Asp.Net Core, filters execution can be done totally depends on MVC Action pipeline. Also, we will produce custom filters moreover in Asp.net Core. Normally, the filter pipeline executed when any action of the MVC Controller needs to be executed or execution already done. There are different types of filters in the Asp.Net Core. Below tables explain the different filter types along with their importance in the process flow.
The below image demonstrates the process flow including execution flow in Asp.Net Core –
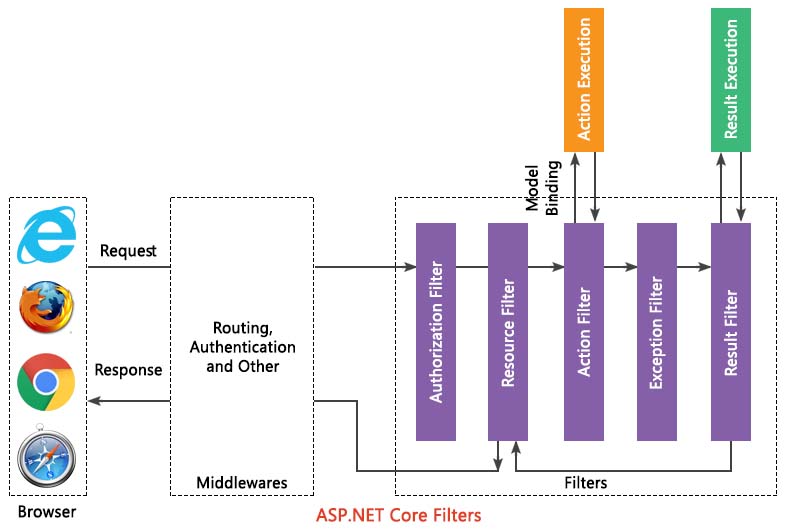
We can implements filter in two different ways. One is the synchronous way and another is the asynchronous way. As from the process name, the process of implementation of these types is totally different. In the case of Synchronous filters, it is normally executed the code before the execution of the action method OnStageExecuting and OnStageExecuted. The example of the Synchronous filters is ActionFilter. The OnActionExecuting technique is termed before the action technique and OnActionExecuted technique is termed once the action technique.
namespace CoreDemoSamples.Filters { public class MyActionFilter : IActionFilter { public void OnActionExecuting(ActionExecutingContext objContext) { } public void OnActionExecuted(ActionExecutedContext objContext) { } } }
On the other hand, Asynchronous filters outline one OnStageExecutionAsync methodology. This type of filter method execution always depends on the FilterTypeExecutionDelegate delegate. As demonstrated in the below code, the ActionExecutionDelegate parameter basically responsible for execution call of the next filter action. Also, we can execute the action method code before the filter executions.
namespace CoreDemoSamples.Filters { public class CustomAsyncActionFilter : IAsyncActionFilter { public async Task OnActionExecutionAsync( ActionExecutingContext objContext, ActionExecutionDelegate objNext) { } } }
FILTER SCOPES AND ORDER OF EXECUTION
In Asp.Net Core, the filters are often side to the pipeline at one in every of three different scopes
by action methodology
by controller category
by global declaration means we can use or apply those filters either in controller level or action method level or both.
If we want to register the filters, then we can define those within the ConfigureServices method with the help MvcOption.Filters.
public void ConfigureServices(IServiceCollection objServices) { objServices.AddMvc(options=> { //an instant options.Filters.Add(new MyActionFilter()); //By the type options.Filters.Add(typeof(MyActionFilter)); }); }
When multiple filters area unit applied to the actual stage of the pipeline, the scope of filter defines the default order of the filter execution. In the filter process flow, the first global level filter is executed first, then it is executed the controller level filters and at last, it will execute the action method level filters. The below image displays the filter process execution order.
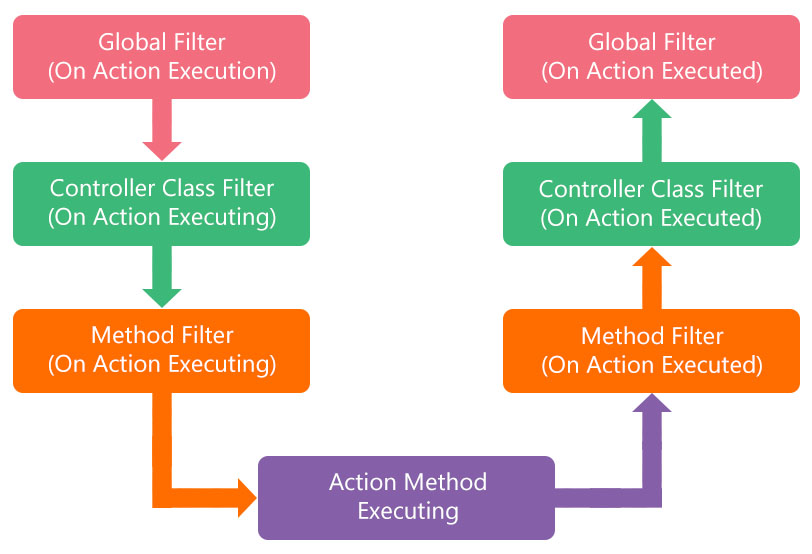
If we want to override the process execution order of the filter, then we can perform that with the help IOrderedFilter interface. This interface has the property named "Order" that use to work out the order of execution. In this case, the process flow normally executes the filters in the ascending sequence of the “Order” means a lower order to higher order. we will set up the order property exploitation the creator parameter.
public class DemoFilterAttribute : Attribute, IActionFilter, IOrderedFilter { public int SequenceNo { get; set; } public void OnActionExecuting(ActionExecutingContext objContext) { //before the action executes } public void OnActionExecuted(ActionExecutedContext objContext) { //after the action executes } }
Now, this DemoFilterAttribute can be applied in the controller action method as below –
[DemoFilter(SequenceNo = 1)] public class UserController : BaseController { public IActionResult HomeIndex() { return View(); } }
In the normal scenario, the order sequence no of all the inbuilt filters are 0. If we want to create any custom filter, then order sequence no of that filter must be stared from 1. Because, at the execution time, it will soften the filter list on the basis of Order and then start execution as per sorted filter list.
FILTER DEPENDENCY INJECTION
Filters will be added by sort or by instance. In the case of the instance, each request is used for this instance. If you add a sort, it'll be type-activated, that means an instance is created for every request and any constructor dependencies are inhabited by dependency injection (DI). Adding a filter by sort is equivalent to the constructor of a class. Filters that area unit enforced as attributes and add on to controller categories or action ways cannot have builder dependencies provided by dependency injection (DI). If we developed some filters which contain dependency, then we need to use that dependency with the help of dependency injection. You can apply your filter to a category or action methodology victimization one in every of the following
ServiceFilterAttribute
TypeFilterAttribute
IFilterFactory
In the case of a ServiceFilter, we can filter instance with the help of Dependency Injection. For activate this filter, we first need to add this filters with the help of ConfigureService and then, we can use the reference of this filter either in the controller class or action method as a ServiceFilter. One of the dependencies we would need to urge from the DI could be a faller among filter, we would log one thing happened. An example is an action filter with faller dependency.
public class DemoFilterForDI : IActionFilter { private ILogger _objLogger; public DemoFilterForDI(ILoggerFactory objFactory) { _objLogger = objFactory.CreateLogger<DemoFilterForDI>(); } public void OnActionExecuting(ActionExecutingContext objContext) { //before the action executes _objLogger.LogInformation("OnActionExecuting"); } public void OnActionExecuted(ActionExecutedContext objContext) { //after the action executes _objLogger.LogInformation("OnActionExecuted"); } }
Now, register this filter in the configuration service method as below
public void ConfigureServices(IServiceCollection objService) { objService.AddScoped<DemoFilterForDI>(); }
Now, use the above service filter in the action method of the controller
[ServiceFilter(typeof(DemoFilterForDI))] public IActionResult Index() { return View(); }
It is terribly kind of like ServiceFilterAttribute and conjointly enforced from IFilterFactory interface. In this case, it does resolve directly from the dependency injection, but it actually initializes the type with the help of "Microsoft.Extensions.DependencyInjection.ObjectFactory". Due to this difference, we need to register types within the ConfigureService method which are already referenced in TypeFilterAttribute. The "TypeFilterAttribute" will optionally settle for builder arguments for the sort. Following example demonstrates the way to pass arguments to a sort victimization TypeFilterAttribute.
[TypeFilter(typeof(DemoFilterAttribute), Arguments = new object)] public IActionResult AboutIndex() { return View(); }